CQRS Architecture
- Stands for Command and Query Responsibility Segregation.
- The central concept of this pattern is that an application has read operations and write operations that must be totally separated.
- This also means that the model used for write operations (commands) will differ from the read models (queries).
- Furthermore, the data will be stored in different locations.
- In a relational database, this means there will be tables for the command model and tables for the read model.
- Some implementations even store the different models in totally different databases, e.g. SQL Server for the command model and MongoDB for the read model.
- This pattern is often combined with event sourcing.
Mechanics
- There are two types of methods in object-oriented software: commands and queries.
- Commands are operations that change the application state and return no data.
- These are the methods that have side effects within the application.
- Queries are operations that return data but don’t change application state.
- In concrete terms for .NET developers, your application will have two “stacks,” or namespaces.
- One namespace will be YourApplication.CommandStack and the other YourApplication.QueryStack.
- This structure gives you a clear picture of what pieces of code change the state of the application.
- If you like to think of software in terms of domain models, this structure has two of them—a query model and a command model.
- These are different models that have their own operations and representations of data.
- These representations can then be optimized for their specific purpose.
- Changes to data tend to cause the most bugs.
- Having a clear understanding of which parts of the application change data and which do not will help with maintainability and debugging.
Workflow
- When a user performs an action, the application sends a command to the command service.
- The command service retrieves any data it needs from the command database, makes the necessary manipulations and stores that back in the database.
- It notifies the read service so that the read model can be updated.
- When the application needs to show data to the user, it can retrieve the read model by calling the read service.
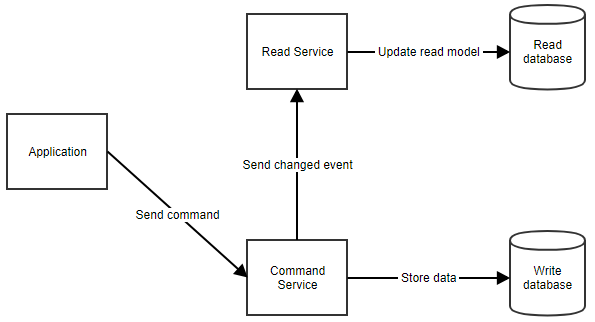
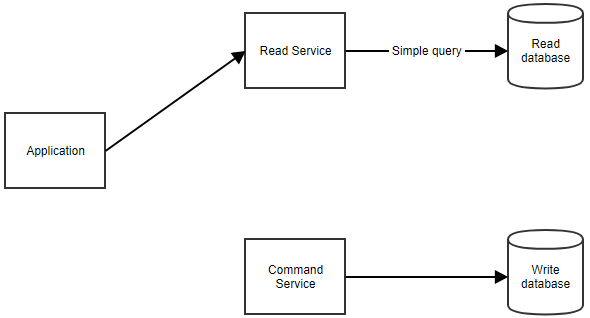
CQRS Principles
Basic CQRS
- Following basic CQRS design allows for optimization of reads and writes.
- The design of the query stack represents the most efficient way to read data.