- In JavaScript, every running function, code block, and the script as a whole have an associated object known as the Lexical Environment.
- The Lexical Environment object consists of two parts:
- Environment Record: an object that has all local variables as its properties (and some other information like the value of
this
).
- A reference to the outer lexical environment, usually the one associated with the code lexically right outside of it (outside of the current curly brackets).
- So, a “variable” is just a property of the special internal object, Environment Record.
- “To get or change a variable” means “to get or change a property of the Lexical Environment”.
- For instance, in this simple code, there is only one Lexical Environment:

- This is a so-called global Lexical Environment, associated with the whole script.
- For browsers, all
<script>
tags share the same global environment.
- On the picture above, the rectangle means Environment Record (variable store) and the arrow means the outer reference.
- The global Lexical Environment has no outer reference, so it points to
null
.
- Here’s the bigger picture of how
let
variables work:
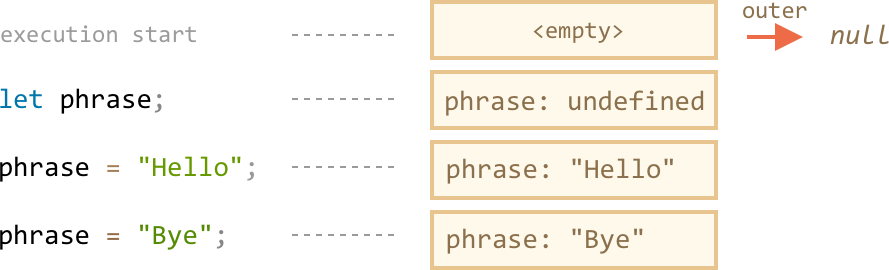
- A variable is a property of a special internal object, associated with the currently executing block/function/script.
- Working with variables is actually working with the properties of that object.
Function Declaration
- Function Declarations are special.
- Unlike
let
variables, they are processed not when the execution reaches them, but when a Lexical Environment is created.
- For the global Lexical Environment, it means the moment when the script is started.
- That is why we can call a function declaration before it is defined.
- The code below demonstrates that the Lexical Environment is non-empty from the beginning.
- It has
say
, because that’s a Function Declaration and later it gets phrase
, declared with let
:
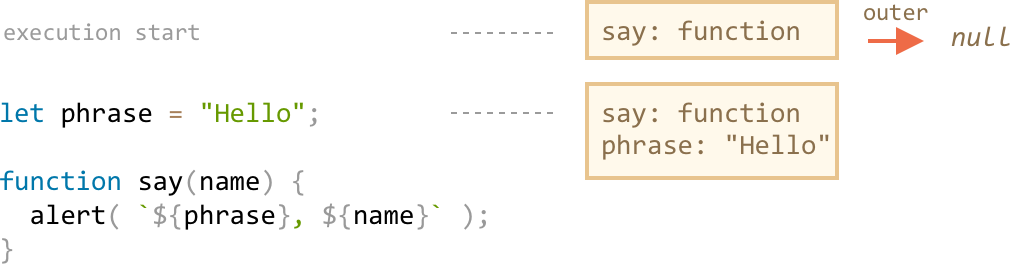